Working with REST API is quite simple and straight forward. For example when you need to fetch data from a list you can use the following JQuery Ajax code snippet:
jQuery.ajax({ url: "http://YourSite/_api/web/lists/getbytitle('ListName')/items", type: "GET", headers: { "Accept": "application/json;odata=verbose" }, success: function(data, textStatus, xhr) { var dataResults = data.d.results; alert(dataResults[0].Title); }, error: function(xhr, textStatus, errorThrown) { alert("error:"+JSON.stringify(xhr)); } });
Another good example is when you need to get specific fields like “Title”, “ID” or “Modified” , you can use the "$select keyword.
For example:
url: "http://YourSite/_api/web/lists/getbytitle('ListName')/items$select= Title ,ID, Modified", type: "GET", headers: { "Accept": "application/json;odata=verbose" }, success: function(data, textStatus, xhr) { var dataResults = data.d.results; alert(dataResults[0].Modified); }, error: function(xhr, textStatus, errorThrown) { alert("error:"+JSON.stringify(xhr)); } });
But what happens when you need to get a user/group field like “Author” ? well, things are not as obvious as they seem.
Unfortunately you can’t use /getbytitle(‘ListName’)/items or/getbytitle(‘ListName’)/items?filter=Author to get the user field since this field does not exist in the response data, but luckily for us we have an “AuthorId” field that (as you already guessed) will get us the user id.
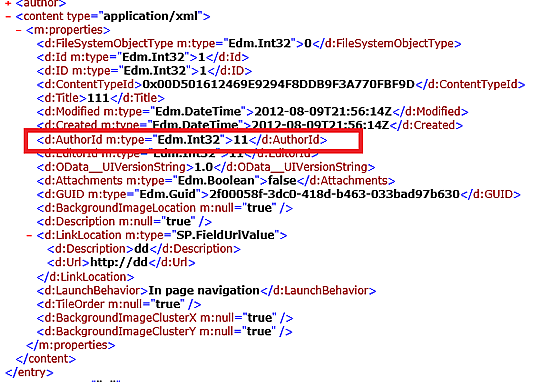
So, after getting the user id from your list you need to make another Ajax call to get the user login name/display name by using /_api/Web/GetUserById method .
Example:
function getUser(id){ var returnValue; jQuery.ajax({ url: "http://YourSite/_api/Web/GetUserById(" + id + ")", type: "GET", headers: { "Accept": "application/json;odata=verbose" }, success: function(data) { var dataResults = data.d; //get login name var loginName = dataResults.LoginName.split('|')[1]; alert(loginName); //get display name alert(dataResults.Title); } }); }
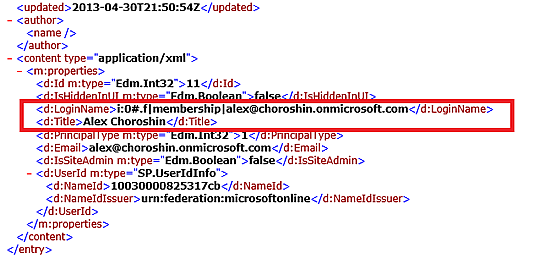
Full Example:
jQuery.ajax({ url: "/SiteName/_api/web/lists/getbytitle('ListName')/items", type: "GET", headers: { "Accept": "application/json;odata=verbose" }, success: function(data, textStatus, xhr) { var dataResults = data.d.results; var resultId = dataResults[0].AuthorId.results[0]; getUser(resultId) }, error: function(xhr, textStatus, errorThrown) { alert("error:"+JSON.stringify(xhr)); } }); function getUser(id){ var returnValue; jQuery.ajax({ url: "http://YourSite/_api/Web/GetUserById(" + id + ")", type: "GET", headers: { "Accept": "application/json;odata=verbose" }, success: function(data) { var dataResults = data.d; //get login name var loginName = dataResults.LoginName.split('|')[1]; alert(loginName); //get display name alert(dataResults.Title); } }); }
No comments:
Post a Comment