We will learn how to acquire Access Tokens for the Dynamics 365 Customer Engagement (CRM) Web API, to perform different operations in Dynamics 365. This will be achieved by using the Microsoft Authentication Library (MSAL) in .NET.
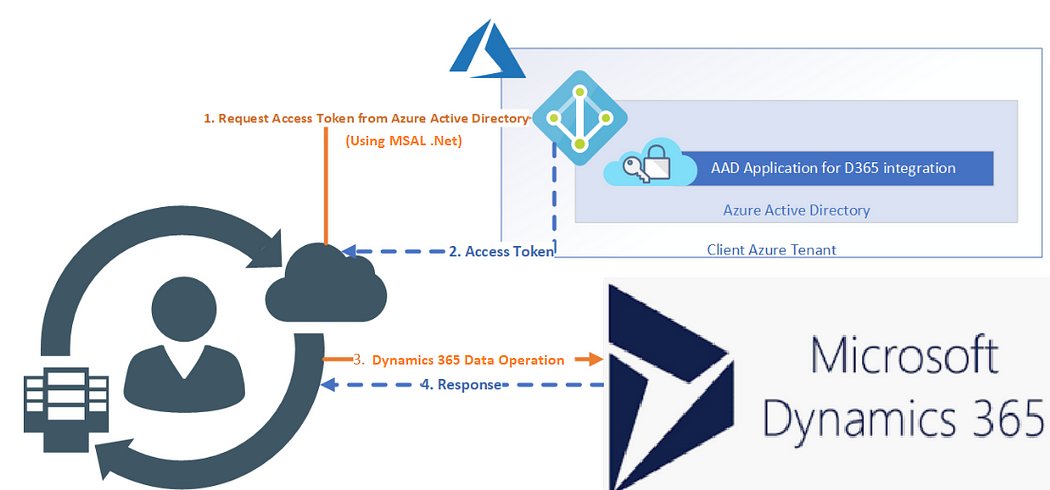
The formerly used ‘CreateFromResourceUrlAsync’ method in the ‘AuthenticationParameters’ class is now obsolete — here is the Microsoft Docs article. A code snippet similar to the below was previously used to obtain an access token for the CRM web API using Azure AD Authentication Library (ADAL).
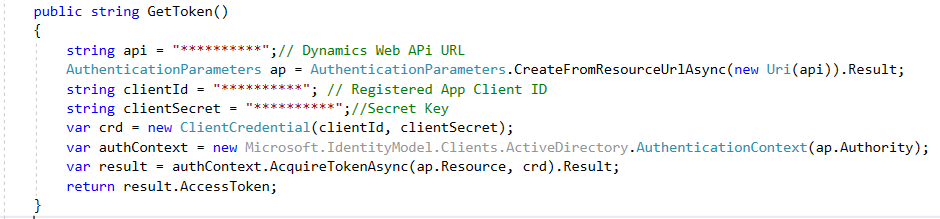
Due to the now obsolete ‘CreateFromResourceUrlAsync’ method, Microsoft recommend using MSAL.Net Authentication Library. Here is the Microsoft Docs article. Later in this post we will explore how to obtain CRM Web API Access tokens using MSAL.Net, removing any dependencies on ADAL.Net.
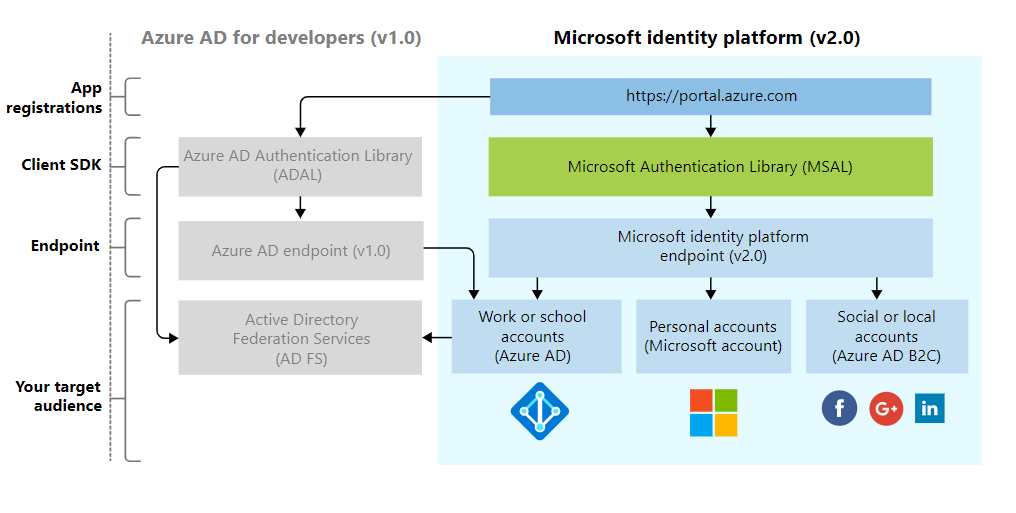
Prerequisites
- Azure Portal Access
- Dynamics 365 Access
- APP Registration Access on Azure AD
- Visual Studio
App Registration and Dynamics 365 API Permission Request in Azure
Whether you are moving your code from ADAL to MSAL, or writing brand new code in MSAL, there is no change in the App Registration and the requesting of Dynamics permissions process. I would however like to highlight a few steps showing how to perform an App Registration and granting of permissions.
- Login to Azure and locate the ‘Azure Active Directory’ service
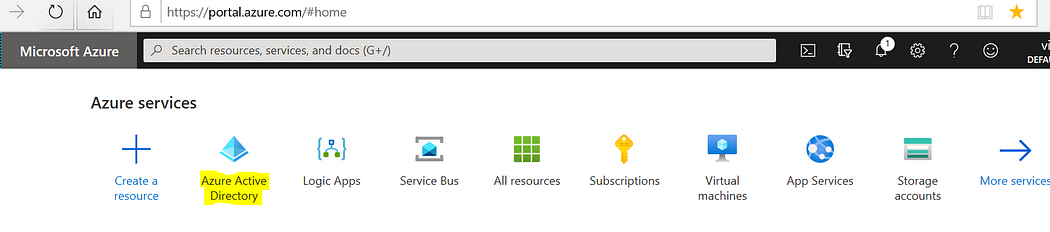
2. Open the ‘Azure Active Directory’ service and click on ‘New Registration’
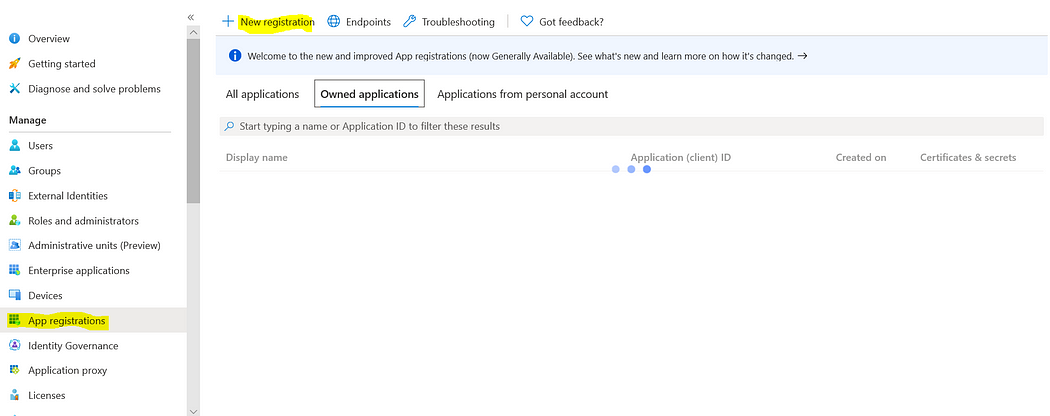
3. Register your app by entering the below details (This is a Single Tenant example)
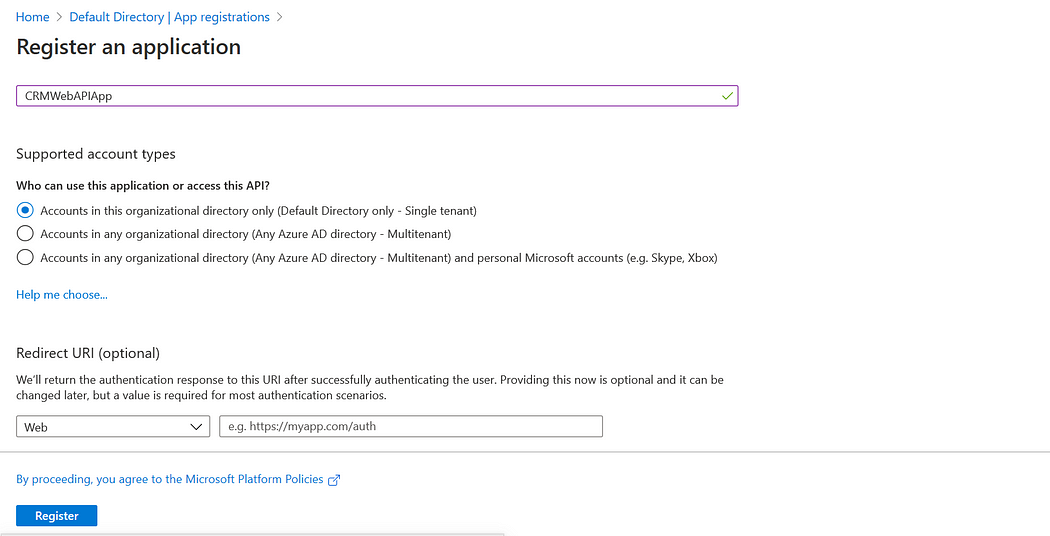
4. Navigate to the ‘API permissions’ section on your registered app. Click on ‘Add a permission’, which will open the ‘Request API permissions’ window (below). Click on ‘Dynamics CRM’ as highlighted.
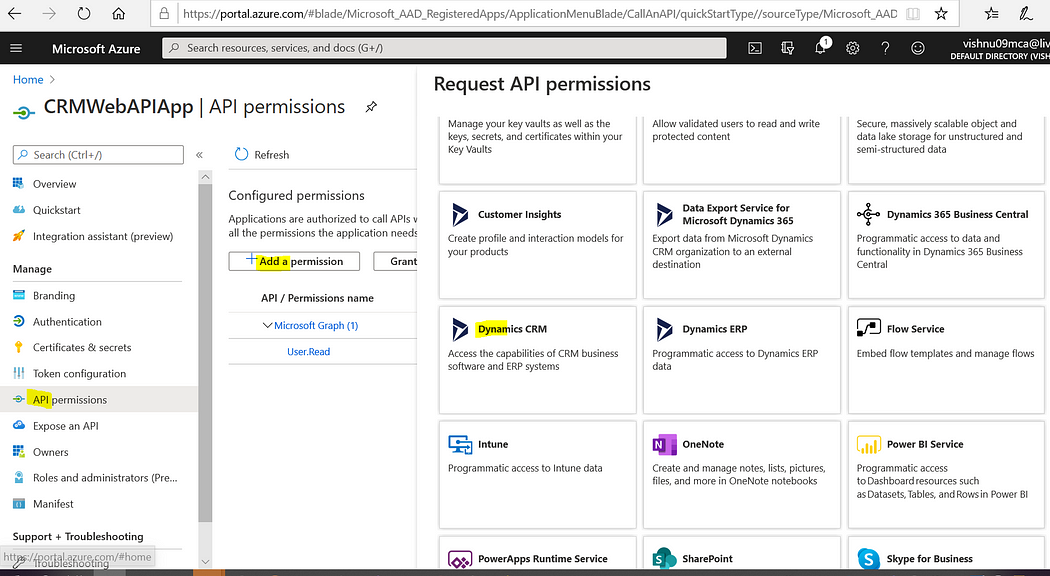
5. Select and add Permissions to your app as below.
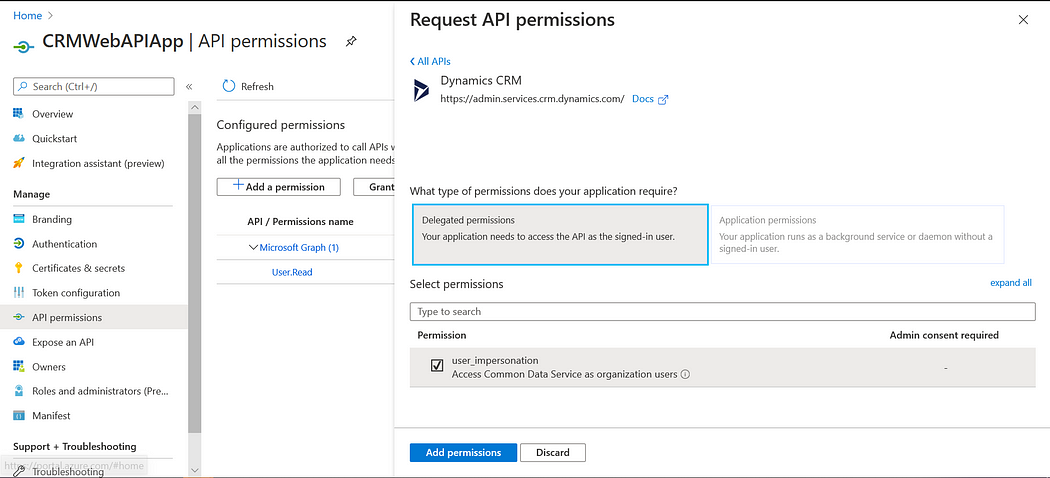
6. Add a Secret Key to your app.
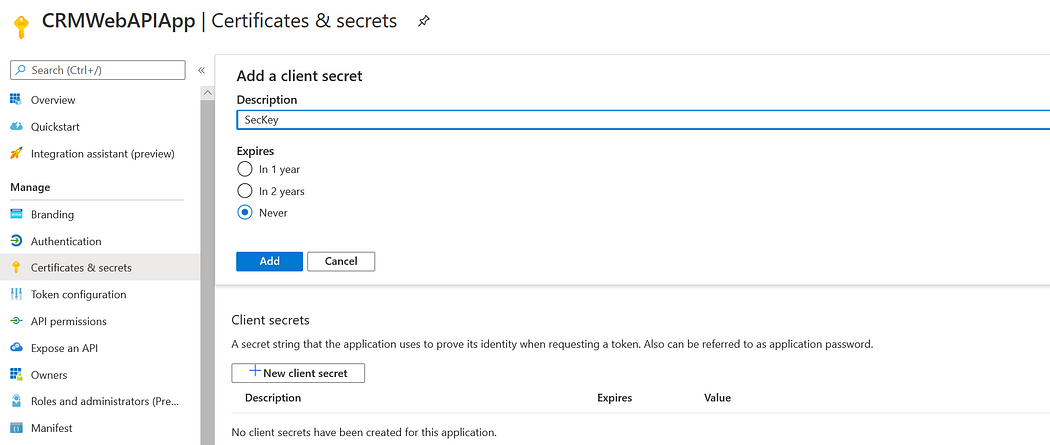
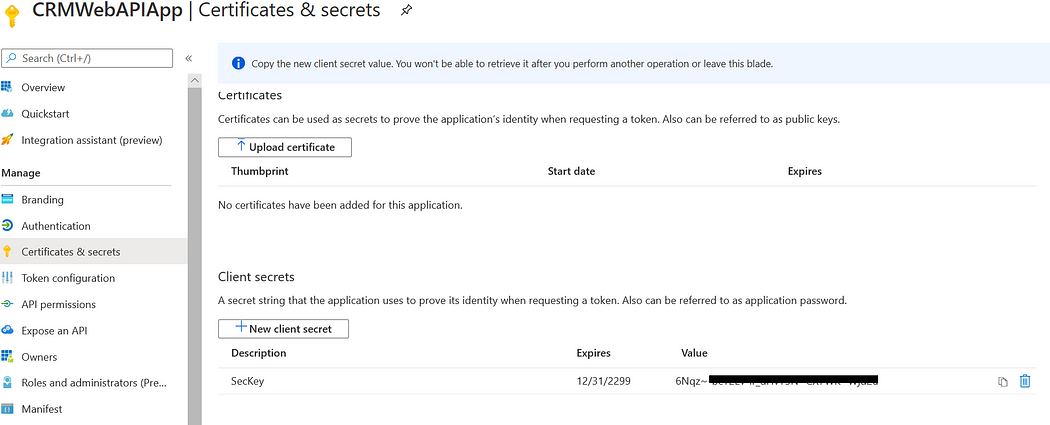
7. Copy the below attributes from your registered app. Each of which will be required in your code to obtain an Access Token.
- Client Secret Key.
- Application (Client) ID.
- Directory (Tenant) ID.
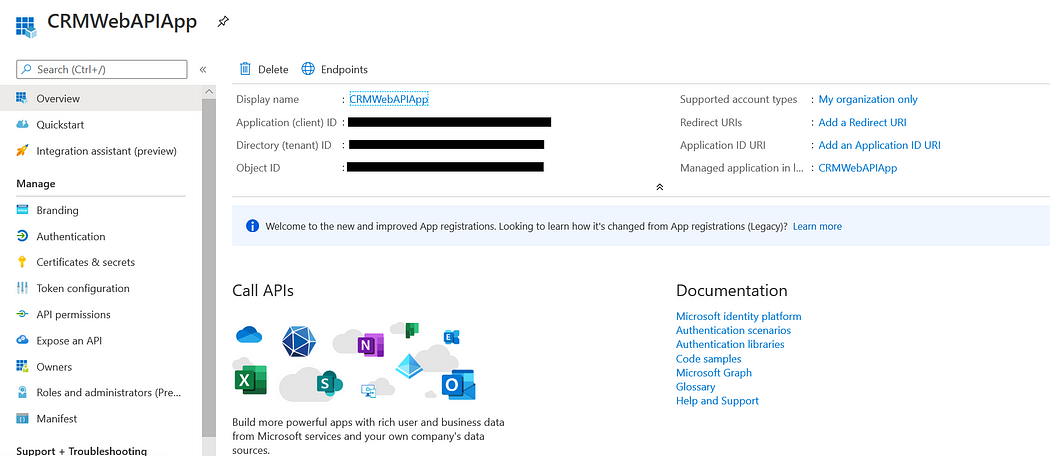
Acquire CRM Web API Token using MSAL.Net
- Create a Visual Studio project and add the NuGet reference of ‘Microsoft.Identity.Client’ as seen below.
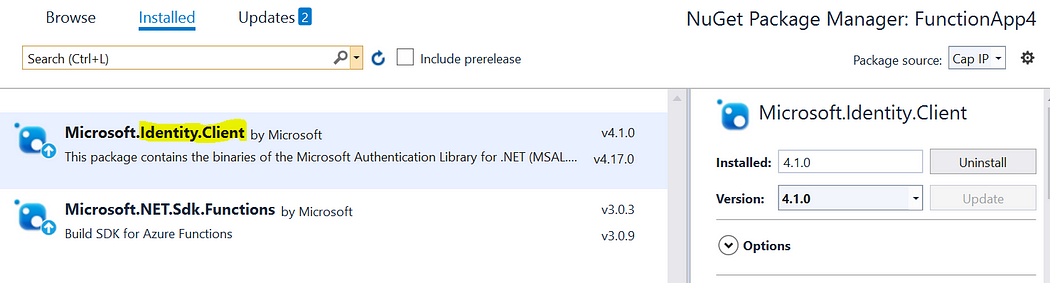
2. We will use the ‘ConfidentialClientApplicationBuilder’ class to acquire a token which is available in the MSAL library class. This class has the below definition.
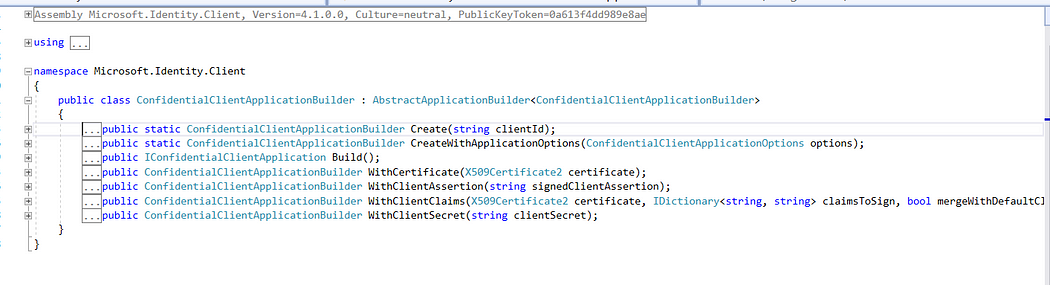
3. Instantiate ‘Confidential Client Application Builder’ using ‘Client Id’, ‘Tenant Id’ and ‘Client Secret key’ — each of which we coped in Step 7 of the previous section.

4. Initialise a scope string array which will be used in acquiring token. In the below snippet, ‘CRM Org URL’ is the URL of your target CRM organisation and will look something like this: https://*.crm*.dynamics.com

5. Last but not least, use the ‘AcquireTokenForClient’ function by passing the ‘scope’ string array and using ‘AuthenticationResult’. This will result in the Access Token and other details being returned.
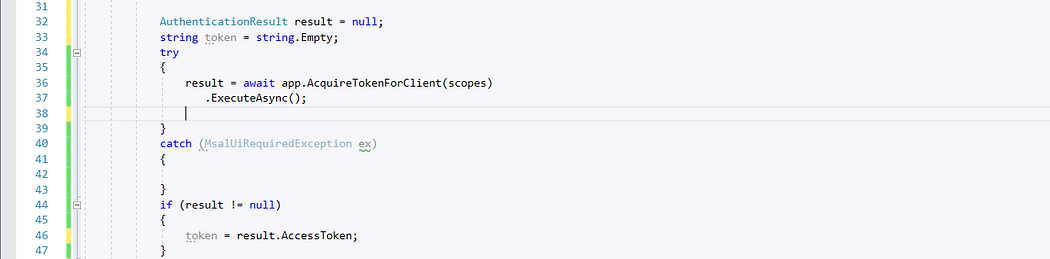
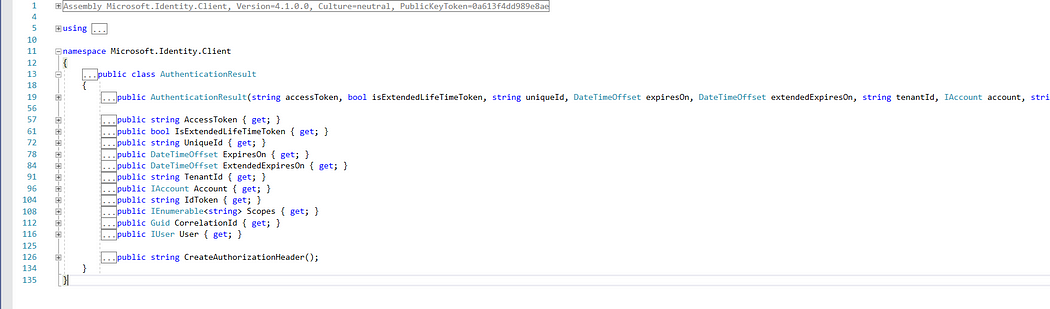
In MSAL.NET, ‘AcquireTokenForClient’ uses the application token cache, while all other ‘AcquireTokenX’ methods use the user token cache. Be sure not to call ‘AcquireTokenSilent’ before you call ‘AcquireTokenForClient’, as ‘AcquireTokenSilent’ uses the user token cache, while ‘AcquireTokenForClient’ checks the application token cache itself and updates it.
Use Token to Perform Operation In CRM
The token generated in the previous section will be used to perform many CRM operations, such as retrieving the top 10 accounts.
No comments:
Post a Comment