Client Object Model :-
Client Object Model is a new feature of SharePoint 2010. It provides features to program against a SharePoint site using .NET Managed Code or JavaScript.
The Client Object Model provides almost all the programming features of the Server Object Model plus advantages in deployment. The Client OM (Client Object Model) is being used as the core programming aid for SharePoint 2010 and thus widely used in the market.
Advantages
- Less Deployment Hassles: Using Client OM, you do not need to install the components required by the Server Object Model. Thus Client OM provides much ease to the end user.
- Language Flexibility: We can use the following languages to work with the Client OM:
- Microsoft .NET
- Silverlight
- ECMA Script (JavaScript /JScript)
- Query Speed Optimizations: In the Client OM, reduced network traffic is attained using Query Optimizations. Thus the user will feel reduced round trips and other advantages like paged results, etc.
How it works?
The Client OM works by sending an XML Request. The server will return a JSON response which is converted to the appropriate Object Model.
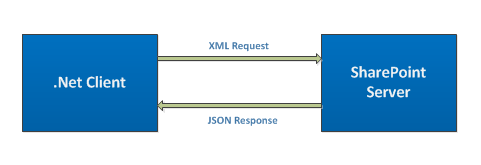
Supported Languages
Following are the programming language/platforms supported for Client Object Model:
- .NET Languages (C#, VB.NET etc.)
- Silverlight
- Scripting Languages (JavaScript, Jscript)
Core Assemblies
There are two assemblies to be referred for working with the Client Object Model.
- Microsoft.SharePoint.Client.dll
- Microsoft.SharePoint.Client.Runtime.dll
These assemblies can be found in the 14 Hive folder: %ProgramFiles%\Common Files\Microsoft Shared\web server extensions\14\ISAPI.
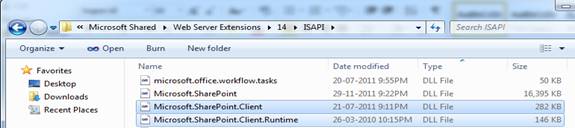
Classes inside Client Object Model
In C#, comparing with classes of the Server Object Model, we can see that Client Object Model has similar classes with a suffix in the namespace and no SP prefix in the class name.
For example:
SPSite
in the Server Object Model is represented in the Client OM as Site
with namespaceMicrosoft.SharePoint.Client
.Client Object Model | Server Object Model |
Microsoft.SharePoint.Client.ClientContext | SPContext |
Microsoft.SharePoint.Client.Site | SPSite |
Microsoft.SharePoint.Client.Web | SPWeb |
Microsoft.SharePoint.Client.List | SPList
|
Add the references Microsoft.SharePoint.dll and Microsoft.SharePoint.Client.dll.
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using Microsoft.SharePoint;
using Microsoft.SharePoint.Client;
namespace CreateListItem
{
class Program
{
static void Main(string[] args)
{
string siteUrl = "http://servername:12345/";
ClientContext clientContext = new ClientContext(siteUrl);
List oList = clientContext.Web.Lists.GetByTitle("
ListItemCreationInformation listCreationInformation = new ListItemCreationInformation();
ListItem oListItem = oList.AddItem(listCreationInformation);
oListItem["Title"] = "Hello World";
oListItem.Update();
clientContext.ExecuteQuery();
}
}
}
Update List Item:
Add the references Microsoft.SharePoint.dll and Microsoft.SharePoint.Client.dll.
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using Microsoft.SharePoint;
using Microsoft.SharePoint.Client;
namespace UpdateListItem
{
class Program
{
static void Main(string[] args)
{
string siteUrl = "http://servername:12345/";
ClientContext clientContext = new ClientContext(siteUrl);
List oList = clientContext.Web.Lists.GetByTitle("
ListItem oListItem = oList.GetItemById(5);
oListItem["Title"] = "Hello World Updated!!!";
oListItem.Update();
clientContext.ExecuteQuery();
}
}
}
Delete List Item:
Add the references Microsoft.SharePoint.dll and Microsoft.SharePoint.Client.dll.
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using Microsoft.SharePoint;
using Microsoft.SharePoint.Client;
namespace UpdateListItem
{
class Program
{
static void Main(string[] args)
{
string siteUrl = "http://servername:12345/";
ClientContext clientContext = new ClientContext(siteUrl);
List oList = clientContext.Web.Lists.GetByTitle("
ListItem oListItem = oList.GetItemById(
oListItem.DeleteObject();
clientContext.ExecuteQuery();
}
}
}
No comments:
Post a Comment